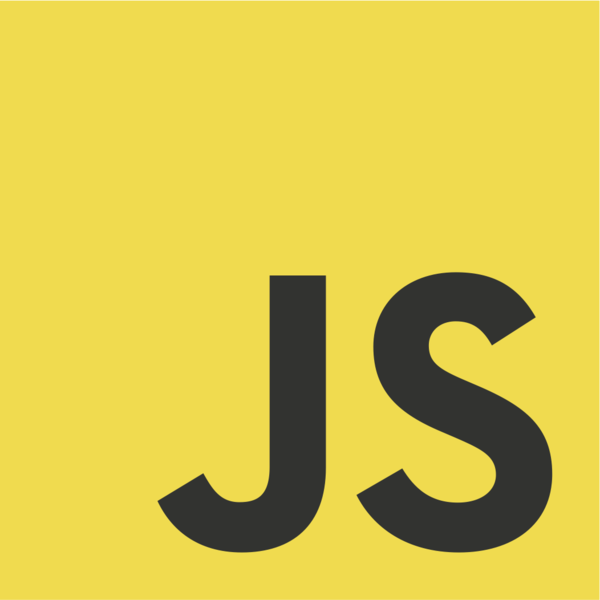
箭頭函式算是 ECMAScript 中常見的用法之一,但他與一般的函式差別在哪?就讓我們一起來瞭解箭頭函式到底是什麼吧。
基礎用法
一般我們在使用函式我們可能會這麼做:
1 | // 一般函式宣告(function declaration)的用法 |
而箭頭函式最主要是將函式運算式或函式陳述式轉化成這種撰寫方式:
1 | const sum = (x, y) => { |
進階用法
省略
當函式語句內部只有運算式的時候還可以省略大括號與宣告返回值的部分:
1 | const sum = (x, y) => x + y |
如果只有一個參數還可以省略中間小括號的部分(如果沒有或有兩個以上的參數就需要小括號):
1 | const greaterThanOne = x => x > 1 |
預設參數(Default parameters)與剩餘函數(Rest parameter)
在箭頭函式中一樣可以使用預設函數與剩餘函數:
1 | const sum = (x = 0, ...y) => { |
this 指向
我們都知道 JavaScript 是採用靜態作用域,而箭頭函式沒有 this 屬性,因此在箭頭函式中的 this 會指向的是父層定義箭頭函式當下的 this:
1 | const ShawnL = { |
若使用箭頭函式的情況:
1 | const name = 'anonymous' |
箭頭函式對於 this 的影響不小,像是在 Vue.js 框架中的 computed 若使用箭頭函式就會因為 this 指向問題而產生錯誤:
1 | new Vue({ |
而 React.js 則是利用了這個特性,在撰寫 class component 簡化寫法:
1 | class CardWrapper extends React.component { |
以上就是箭頭函式的用法,我自己是還蠻常用到的,使用時要注意的就是瀏覽器支援度與 this 指向問題,確認沒問題後箭頭函式將會大幅提升程式碼可閱讀性與開發速度。